https://nextjs.org/learn/basics/create-nextjs-app
Learn | Next.js
Production grade React applications that scale. The world’s leading companies use Next.js by Vercel to build pre-rendered applications, static websites, and more.
nextjs.org
저번에 이어서, 오늘은 Assets, Metadata, and CSS 부분을 정리해보자.
1. Assets
HTML에서 이미지를 불러오는 방식과는 달리, Next.js는 기본적으로 이미지 최적화를 지원한다.
//HTML
<img src="/images/profile.jpg" alt="Your Name" />
//Next.js
import Image from 'next/image'
const YourComponent = () => (
<Image
src="/images/profile.jpg" // Route of the image file
height={144} // Desired size with correct aspect ratio
width={144} // Desired size with correct aspect ratio
alt="Your Name"
/>
)
(저번에도 그렇고 import를 많이 사용하는 것 같음)
2. Metadata - <title> 수정하기
<title>은 <head>의 일부이므로, <head> 수정하는 방법을 알아보자.
index.js
<Head>
<title>Create Next App</title>
<link rel="icon" href="/favicon.ico" />
</Head>
<head>대신에 <Head> 즉, 대문자를 사용한다. <Head>는 Next.js에 내장된 React Component이다. 'next/head' 모듈에서 'Head' component를 import 할 수 있다.
앞에서 first-post.js에 <title>을 추가하지 않았다. 먼저 import를 한 후, 추가해보자.
import Link from 'next/link'
import Head from 'next/head' //추가된 부분
export default function FirstPost() {
return (
<>
<Head> //추가된 부분
<title>First Post</title> //추가된 부분
</Head> //추가된 부분
<h1>First Post</h1>
<h2>
<Link href="/">
<a>Back to home</a>
</Link>
</h2>
</>
)
}
개발자 도구에서 <title>이 생성 된 것을 확인 할 수 있다.
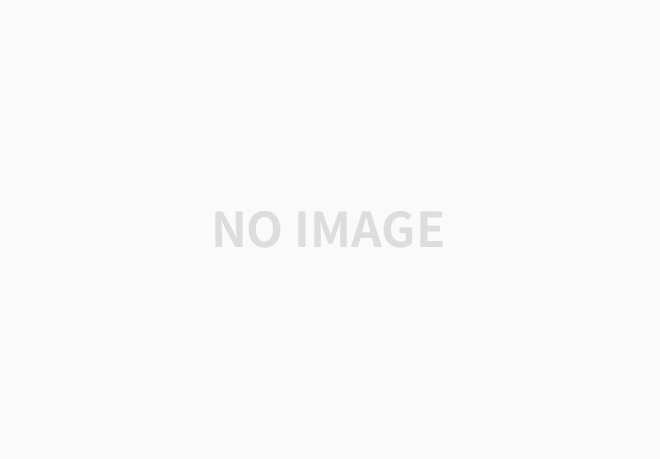
3. JavaScript 추가(타사)
일반적으로 하는 것 처럼, <Head>에 <script>요소를 사용해 외부 스크립트를 추가해도 되지만, 이렇게 한다면 동일한 페이지에서 가져온 다른 JavaScript 코드와 관련하여 로드될 시기를 명확하게 알 수 없다. 따라서 Script Component를 사용한다.
first-post.js
import Link from 'next/link'
import Head from 'next/head'
import Script from 'next/script' //추가된 부분
export default function FirstPost() {
return (
<>
<Head>
<title>First Post</title>
</Head>
//Script 부분 추가됨
<Script
src="https://connect.facebook.net/en_US/sdk.js"
strategy="lazyOnload"
onLoad={() =>
console.log(`script loaded correctly, window.FB has been populated`)
}
/>
<h1>First Post</h1>
<h2>
<Link href="/">
<a>Back to home</a>
</Link>
</h2>
</>
)
}
Script Component 정리
src : 주소
strategy : 스크립트를 로드해야 하는 시기를 제어함, lazyOnload는 특정 스크립트를 브라우저 idle 시간동안 느리게 로드하도록 한다.
onLoad : 스크립트 로드가 완료된 직 후 JavaScript 코드를 실행하는 데 사용된다. 여기서는 스크립트가 올바르게 로드되었다는 메시지를 콘솔에 기록한다.
4. CSS Styling
이미 index.js에는 스타일이 적용되어 있는데, 이는 다음과 같은 코드에서 확인할 수 있다.
<style jsx>{`
…
`}</style>
1. 라이브러리 사용
styled-jsx라는 라이브러리를 사용하고 있다. 이것은 CSS-in-JS 라이브러리이다. 이것을 사용하면 React 구성 요소 내에서 CSS를 작성할 수 있고, 범위가 지정된다.(다른 구성 요소는 영향을 받지 않음). Next.js에는 styled-jsx에 대한 지원이 내장되어 있지만, 다른 라이브러리를 사용할 수 있다.
2. Layout component
모든 페이지에서 공유할 Layout component를 만든다. 'component'라는 폴더를 만들고, 그 안에 layout.js라는 파일을 만든다.
export default function Layout({ children }) {
return <div>{children}</div>
}
그 후, first-post.js에 'Layout'을 import한 후 바깥 구성요소로 만든다.
import Head from 'next/head'
import Link from 'next/link'
import Layout from '../../components/layout'
export default function FirstPost() {
return (
<Layout>
<Head>
<title>First Post</title>
</Head>
<h1>First Post</h1>
<h2>
<Link href="/">
<a>Back to home</a>
</Link>
</h2>
</Layout>
)
}
이제 'Layout' component에 맞게 스타일을 추가한다. components폴더에 layout.modules.css 파일을 만든다.
.container {
max-width: 36rem;
padding: 0 1rem;
margin: 3rem auto 6rem;
}
'contatiner'내부 'components/layout.js'에서 사용하기 위해서는, css 파일을 가지고 오고, styles.contatiner를 className으로 사용한다.
import styles from './layout.module.css'
export default function Layout({ children }) {
return <div className={styles.container}>{children}</div>
}
결과물

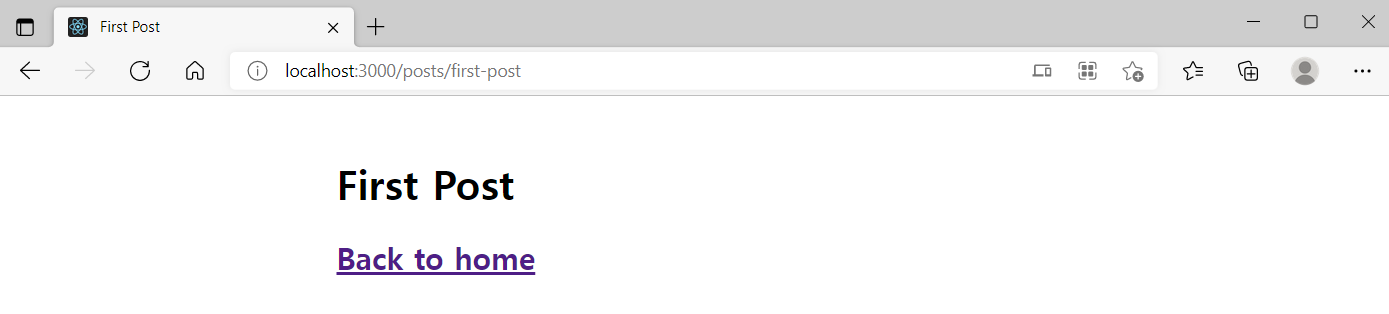
5. Global Styles
일부 CSS를 모든 페이지에서 로드하려면 Next.js가 이것을 지원한다. Global CSS파일을 로드하려면 page/_app.js라는 파일을 만든다.
export default function App({ Component, pageProps }) {
return <Component {...pageProps} />
}
여기서 App component는 다른 모든 페이지에서 공통적으로 사용되는 최상위 구성 요소이다.
global CSS 파일을 page/_app.js에서 가져와 추가할 수 있다. global CSS는 다른 곳에서 가져올 수 없다. 그 이유는, global CSS가 페이지의 모든 요소에(의도하든, 의도하지 않든) 영향을 미치기 때문이다. global CSS 파일을 원하는 위치에 놓고 원하는 이름을 사용할 수 있다. 여기서는 최상위 'styles' 폴더를 생성하고, 'global.css'를 생성했다.
마지막으로 page/_app.js에 css파일 추가 가져오기를 연다.
import '../styles/global.css'
export default function App({ Component, pageProps }) {
return <Component {...pageProps} />
}
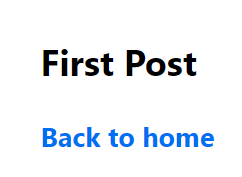
그 이후는 생략!
'Web Service > React' 카테고리의 다른 글
[영화 웹 서비스 만들기] #1. 바닐라JS와 리액트JS의 비교 (0) | 2022.03.14 |
---|---|
[nextjs-blog] #3. Pre-rendering and Data Fetching (0) | 2022.01.11 |
[nextjs-blog] #1. Navigation (0) | 2022.01.08 |
[to-do-list] #3. CRUD (0) | 2022.01.06 |
[to-do-list] #2. 로그인/로그아웃 (0) | 2022.01.06 |
댓글